How to use PHP variables in jQuery in WordPress
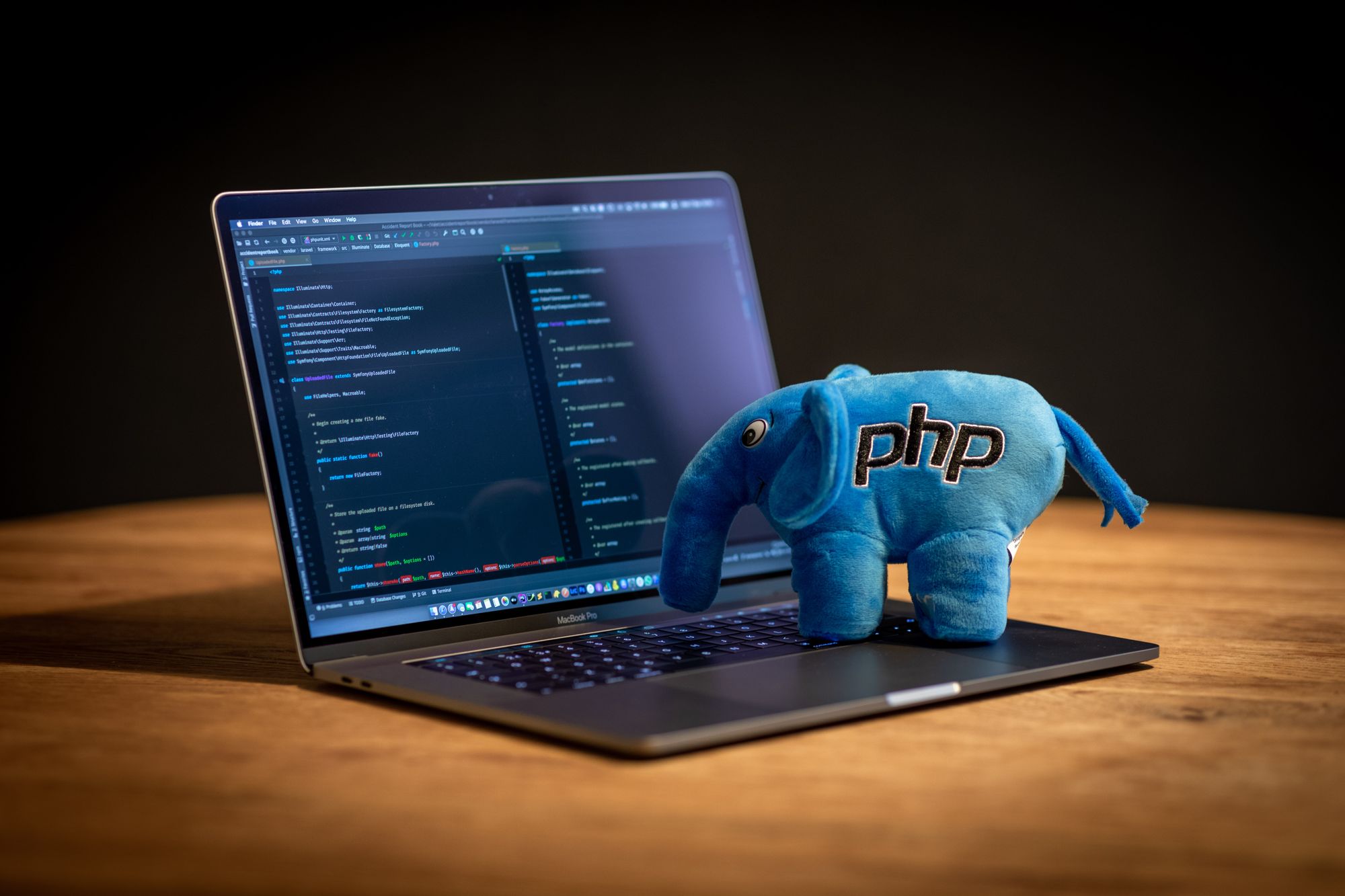
Often times you need access to server-side data in your JavaScript. Most people accomplish this in one of three ways:
- Echo out JavaScript in PHP
- Place data in HTML attributes for jQuery to grab
- Make Ajax request to PHP
I won’t go into the pros and cons of each, but there is actually a fourth way that’s much leaner and cleaner, but unfortunately not a lot of people know about it. In one word: wp_localize_script
.
Here is a step by step of how it works.
Register your script
First things first. You have to tell WordPress that you have a JavaScript file that you want to use at some point. You can do that by registering your script using the wp_register_script
function:
wp_register_script(
'sample-script-name',
plugins_url('scripts/sample-script-name.js', dirname(dirname(__FILE__))),
array('jquery')
);
- Give it a name
- Provide the path to the file
- Indicate any dependencies by name
It’s a good idea to do this early on, when you initialize your plugin, for example, so that the script is ready for you at any point.
Enqueue your script
Now that WordPress knows about your script, you’ll want to enqueue on whatever pages need it. You can do that using the wp_enqueue_script
function:
wp_enqueue_script(
'sample-script-name'
);
- Same script name from step 1.1
The nice thing about this is that WordPress will make sure that any dependencies you specified in 1.3 are enqueued, as long as those scripts have been previously registered.
Localize your script
Chances are that up until now, the previous two steps were nothing new, but here is where the magic begins. Once you’ve enqueued your script, WordPress can replace “placeholders” in your script with real data using the wp_localize_script
function.
Let’s say you need the path to admin-ajax.php
and another piece of custom data in your JavaScript. Here is how you would make that available:
wp_localize_script(
'sample-script-name',
'sample_object_name',
array(
'admin_url' => admin_url('admin-ajax.php'),
'another_variable' => get_some_variable_value()
)
);
- Same script name from step 1.1 and 2.1
- Provide it with a custom object name
- Create an array where the array key is the variable and the array value the data
You can then reference this data in your JavaScript like so:
var admin_url = sample_object_name.admin_url;
var another_variable = sample_object_name.another_variable;
You don’t really have to assign those values to a new variable, but I usually do that in case I want to change my object name later. An important note is that the object name has to be unique per page, so pick something long and custom that only you might use.
Final Note
Although I documented this as three steps, to me, enqueue and localize belong together. Chances are if you’re using both, you don’t want one without the other, which is why I call the wp_register_script
function and then create a custom function called enqueue_localize_sample_script_name
that calls enqueue and localize back to back.
Mind blown, right?
If you have any questions about this or an improvement, feel free to leave a comment below.
Featured image by Ben Griffith.