Remove post type slug in custom post type URL and move subpages to website root in WordPress
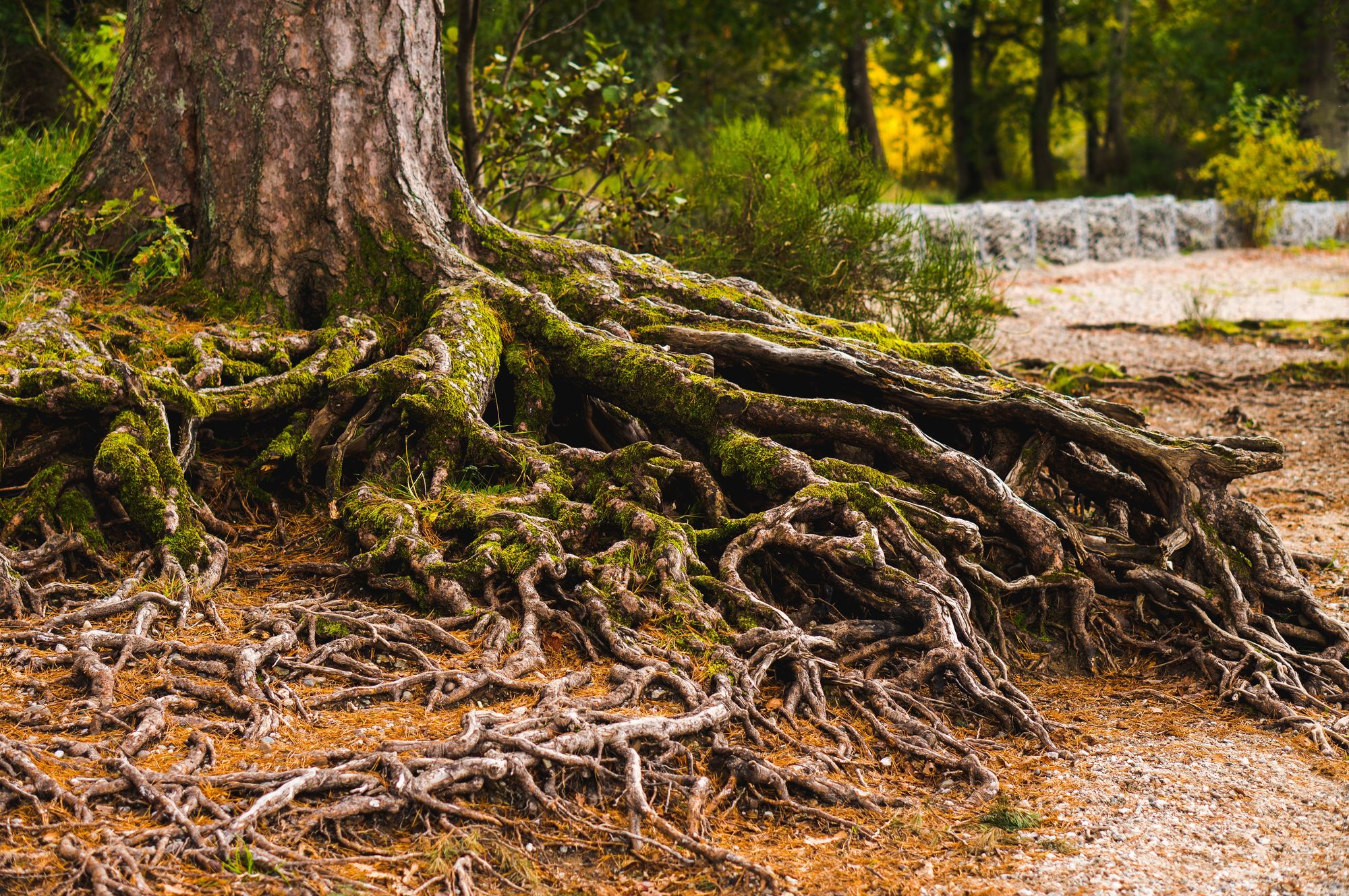
I recently had a need to rewrite the URLs of all parent and child pages in a custom post type so that they appeared to live at the website root, but in reality, continued to live in a custom post type within their hierarchy.
Preface
The situation:
- I have a page called
Services
that lives atdomain.com/services
. - I have a custom post type called
Services
. - I have
Services
post calledProgramming
that lives atdomain.com/services/programming
. - I have
Services
post calledWeb Development
, that is a child ofProgramming
, that lives atdomain.com/services/programming/web-development
.
The goal:
- The
Services
page should remain where it is i.e.domain.com/services
. - The
Programming
post should appear to live at the website root i.e.domain.com/programming
. - The
Web Development
service should also live at the website root i.e.domain.com/web-development
.
The reason:
- Shorter URLs.
- Keep all website pages together.
- Keep all services together.
- Maintain hierarchy of services.
Setup
I have a custom post type setup like so:
function register_services() {
$arguments = array(
'label' => 'Services',
'public' => true,
'capability_type' => 'page',
'hierarchical' => true,
'supports' => array('title', 'editor', 'page-attributes'),
'has_archive' => false,
'rewrite' => true
);
register_post_type('services', $arguments);
}
Solution
Remove “services” from the custom post type URL
We’re going to use the post_type_link
filter to do this.
Update 1/19/14: Tweaked function to work with WordPress installed in a subdirectory.
add_filter(
'post_type_link',
'custom_post_type_link',
10,
3
);
function custom_post_type_link($permalink, $post, $leavename) {
if (!gettype($post) == 'post') {
return $permalink;
}
switch ($post->post_type) {
case 'services':
$permalink = get_home_url() . '/' . $post->post_name . '/';
break;
}
return $permalink;
}
- Line 9: If
$post
is a valid post object. - Line 13: If post belongs to a post type that we want to remove the post type slug from.
- Line 15: Build a new permalink from home URL with just the post name.
All of this will essentially give us URLs like this:
domain.com/programming/
domain.com/web-development/
But neither of those will work – you’ll get a 404 error – because WordPress can no longer identify those posts as Services
posts and therefore attempts to find pages called Programming
and Web Development
, which don’t exist, hence the 404 error.
If you take a look at WordPress’ rewrite rules:
echo '<pre>' . print_r(get_option('rewrite_rules'), true) . '</pre>';
You’ll see something similar to this:
Array
(
[services/(.+?)(/[0-9]+)?/?$] => index.php?services=$matches[1]&page=$matches[2]
[(.?.+?)(/[0-9]+)?/?$] => index.php?pagename=$matches[1]&page=$matches[2]
)
As you can see, using the services
slug, WordPress knows to take whatever comes after it ((.+?)
) and pass it in as the pagename
value ($matches[1]
), which translates to something like:
domain.com/index.php?services=programming
Since the first rewrite rule no longer applies (slug removed), it now passes programming
in as the pagename
without specifying the post type, and since this page doesn’t exist, WordPress can’t return the page.
Add post type back to the post query
Now that WordPress doesn’t know that it’s dealing with a custom post type, we need to provide it with this information. For that we’re going to use the pre_get_posts hook.
add_action(
'pre_get_posts',
'custom_pre_get_posts'
);
function custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
$post_name = $query->get('pagename');
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
switch($post_type) {
case 'services':
$query->set('services', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
}
- Line 7: We’ll need the WordPress database object to get additional information about the post.
- Line 9-11: We’re going to make sure that the current query is the main query. Since a page can have multiple queries, we’re limiting our filter to only the query that gets information about the page itself.
- Line 13: We’re going to grab the
pagename
from the query, which might beprogramming
orweb-development
. - Line 15-20: Now we’re going to use the
pagename
to look in the database for that particular post and extract its post type. - Line 22-23: If this post has a post type that we know we need to manipulate, we’ll need to perform additional actions.
- Line 24: We need to a create a query var for the post type and set it to the
pagename
, so WordPress knows where to actually find the data for the post. - Line 25: We also need to set the
post_type
back toservices
. - Line 26: In order for WordPress to load the right template i.e.
single-services.php
, we need to setis_single
totrue
. - Line 27: Last, but not least, we set
is_page
tofalse
for good measure, since it’s a custom post type.
Update 9/20/13: Changed add_filter('pre_get_posts', 'custom_pre_get_posts');
to add_action('pre_get_posts', 'custom_pre_get_posts');
Update: 12/23/14: As of WordPress 4.0, the code above doesn’t work. The following changes need to be made:
$result = $wpdb->get_row(
$wpdb->prepare(
'SELECT wpp1.post_type, wpp2.post_name AS parent_post_name FROM ' . $wpdb->posts . ' as wpp1 LEFT JOIN ' . $wpdb->posts . ' as wpp2 ON wpp1.post_parent = wpp2.ID WHERE wpp1.post_name = %s LIMIT 1',
$post_name
)
);
switch($post_type) {
case 'services':
if ($result->parent_post_name !== '') {
$post_name = $result->parent_post_name . '/' . $post_name;
}
$query->set('services', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
Result
You’ve met all your goals. In addition, if you try to create a page with the same name as one of your services, you’ll notice that the slug
(i.e. post_name
, pagename
, etc.) will now increment, avoiding a possible collision.
Here are a couple of things to keep in mind:
- I didn’t test the performance of this yet, but if you use some kind of caching system on your website, you should be fine.
- All my functions live within a PHP namespace, so I only prefixed them with
custom_
for simplicity of this post. - I’ve looked at many different solutions online, and they all had their fair share of problems, so this may too, I just don’t know it yet.
If you have questions or suggestions, leave them in the comments below.
Featured image by Eilis Garvey.
Comments (95)
Previously posted in WordPress and transferred to Ghost.
Tristan C
May 29, 2013 at 9:15 am
Thanks for the post! Unfortunately I can’t get it to work… I keep on getting 404s. Have tried updating the permalinks, flush_rewrite_rules();
, etc.
Ryan Sechrest
May 29, 2013 at 12:50 pm
First, I would output the current WordPress rewrite rules (example in post), just to make sure the ones you added are indeed active. Second, I would print out the$query
variable (print_r($query, true)
) incustom_pre_get_posts
to see that the values are set to what you expect (and also make sure the code in the filter is executed).
Marco Panichi
July 7, 2013 at 8:34 am
Hi Ryanm thank you very much for this article. I’m going mad with this slug!!! I’ve tried your solution but it doesn’t work. Maybe you can help me – I have this situation:
– a custom type called “static”
– it’s hierarchical
– my permalink structure is: “/blog/%postname%”
– WordPress 3.5.2
Following your instruction everything works in admin side: I can create a “static” page called “My Page Title” and the permalink in the editor becomes “http://www.—.com/my-page-title”
Unfortunally, in the website I can reach this page only with the URL “http://www.—.com/blog/static/my-page-title”
I have tryed lots of solutions for DAYS!
Ryan Sechrest
July 11, 2013 at 8:36 am
When you go to the permalink that it’s supposed to be, what do you get? A page not found?
GG
July 8, 2013 at 3:34 am
Man… as much as I want this to work its just not happening.
I’m trying to use this in combination with 301 redirects to create a short urls post type.
I didnt have the postype setup to begin with, so it took a minute to figure out I needed to add:add_action( 'init', 'register_services' );
May want to note this in the post.
Also, settinghas_archive
totrue
automatically adds it to your permalink structure, you showfalse
.
My custom permalink structure is set to:/blog/%postname%/
So I was getting/blog/posttype/postname/
Shouldnt have mattered because you’re grabbing the last part of the path, but I think something was screwy there because when I print$post_name
later incustom_pre_get_posts
, it wasn’t finding anything.
Last resort, i think ill use http://wordpress.org/plugins/custom-permalinks/ and change them manually.
Thanks for trying though! Glad it worked for you.
Ryan Sechrest
July 11, 2013 at 8:48 am
Let’s systematically troubleshoot this. For step #1, let’s confirm thepost_type_link
filter is working. If you go anywhere in your template and useget_permalink($id)
, with$id
belonging to aservices
post, please provide the absolute path it printed out (you can omit the domain).
David
July 14, 2013 at 11:43 pm
To get this to work I had to change:$query->set('services', $post_name);
to:$query->set('name', $post_name);
AJ Clarke
July 21, 2013 at 5:24 pm
Works great, thanks man!
kevin
July 30, 2013 at 8:16 pm
What are your thoughts about getting things running with WPMU, as of right now it would default the URL back todomain.com/services/
rather thandomain.com/username/services/
Cheers
kc
Ryan Sechrest
July 31, 2013 at 9:30 pm
You have a couple options.
(1) You could wrap the filters in ais_main_site()
check, which would only apply it to your main site, leaving your child sites as-is.
(2) In step 1, where you remove the “services” slug, you could perform ais_multisite()
check and then parse$post_path
accordingly, essentially accounting for the site name by keeping it in there or putting it back.
Tomas
August 7, 2013 at 8:36 am
Will this work with WPML, too?
I’ve tried this plugin http://wordpress.org/plugins/remove-slug-from-custom-post-type/ but it only removes the slug for the main language…
Ryan Sechrest
August 7, 2013 at 8:55 am
I do have MU enabled on the site that I did this on, however, I’m only applying this code on the main site and not child sites, since don’t need it (yet). Looking at the code above, there is nothing to suggest that it wouldn’t work, except for, as Kevin pointed out, you may have to adjust the $permalink
that’s created in step #1 when MU is installed as sub-directories instead of sub-domains, since there’ll be an extra component in the URL that needs to be accounted for.
Stefan
September 19, 2013 at 2:38 am
Ryan, your tutorial was very useful. My case was similar to yours and I was able to remove the slug. But I made a few modifications for this to work as you can see here https://gist.github.com/stefanbc/6620151. First of all, this line
add_filter('pre_get_posts','custom_pre_get_posts');
should be something like this
add_action('pre_get_posts','custom_pre_get_posts');
because according to the WordPress codex there is no filter pre_get_posts
And another thing I modified was this
$post_name = $query->get('pagename');
to this
$post_name = $query->get('name');
This was my case. Thanks for the awesome tutorial.
Ryan Sechrest
September 20, 2013 at 10:03 pm
Thanks for the feedback, Stefan. You are right about it being an action and not a filter. I’ve updated my post above. With regard topagename
vsname
, if your custom post type is based on a post, which is the default,name
would be in fact the way to go, but if it’s based on a page, I thinkpagename
is recommended. I’m actually using it as a page, but omitted that in my setup– thanks for catching that!
Dennis
October 13, 2016 at 11:49 am
@stefan
Your github code worked except that it was throwing a php error regarding variables. I changed:
$post_name = end(explode('/', trim($post_path, '/')));
to
$path_base = explode( get_template(), str_replace( '\\', '/', dirname( __FILE__ ) ) );
$post_name = ltrim( end( $path_base ), '/' );
and it worked beautifully.
Thanks
chụp ảnh cưới
October 12, 2013 at 4:25 am
I using
[product-category/(.+?)/feed/(feed|rdf|rss|rss2|atom)/?$] => index.php?product_cat=$matches[1]&feed=$matches[2]
[product-category/(.+?)/(feed|rdf|rss|rss2|atom)/?$] => index.php?product_cat=$matches[1]&feed=$matches[2]
[product-category/(.+?)/page/?([0-9]{1,})/?$] => index.php?product_cat=$matches[1]&paged=$matches[2]
[product-category/(.+?)/?$] => index.php?product_cat=$matches[1]
[product-tag/([^/]+)/feed/(feed|rdf|rss|rss2|atom)/?$] => index.php?product_tag=$matches[1]&feed=$matches[2]
[product-tag/([^/]+)/(feed|rdf|rss|rss2|atom)/?$] => index.php?product_tag=$matches[1]&feed=$matches[2]
[product-tag/([^/]+)/page/?([0-9]{1,})/?$] => index.php?product_tag=$matches[1]&paged=$matches[2]
[product-tag/([^/]+)/?$] => index.php?product_tag=$matches[1]
with htaccess?
Ryan Sechrest
October 12, 2013 at 3:58 pm
The rewrite rules I showed were only a subset of those in the system to explain that particular point. What you have works just as well.
Sam
October 30, 2013 at 8:21 am
Sorry for asking – I couldn’t get where do I make the changes :$
Help? O:-)
Ryan Sechrest
October 30, 2013 at 8:38 am
You could put this in your theme’s functions.php
file, however it would probably be best suited in a plugin. Take a look at the WordPress codex on how to create a plugin.
efix
December 11, 2013 at 4:30 pm
Hi,
Can I use your way for post-type pages also? I want to rewrite my URL structure from http://www.ex1.com/parentpage/childpage to http://www.ex1.com/childpage. So your code should work for me to. But it didn’t. Any idea why?
thank you
efix
Ryan Sechrest
December 12, 2013 at 2:40 am
This code will not work on native post types, only custom post types.
Victor
December 30, 2013 at 3:59 pm
here is how i did:
register_post_type with these args'rewrite' = array('slug' => '/','with_front' => false)
'capability_type' => 'page'
using this approach there is no need to usepost_type_link
filter
then comescustom_pre_get_posts
Ryan Sechrest
December 30, 2013 at 9:27 pm
Thanks for sharing, Victor. I’ll try that out next time I’m working with that code!
Bill
January 12, 2014 at 10:55 am
Great article. THis seems to work for me on my sandbox site except one thing….
I have a main wordpress website at http://www.website.com, but I have an additonal wordpress install in a folder of the main website….so in other words, I have my live site here: http://www.website-name.com
but I installed another wordpress install in a folder I named DEMSO off that live site I use for sandbox stuff. http://www.website-name.com/demos/SANDBOX-SITE
I never have any problems, however when I am trying to use this code on the sandbox site, when I view the custom post type, it takes me back to the root wordpress install, so instead of the custom post type url being
http://www.website-name.com/demos/SANDBOX-SITE/post-name (the CPT slug is gone…yeah!!)
it is http://www.website-name.com/post-name
Can you recommend the code alteration so it doesnt strip it all the way back to the main website root, and instead takes it back to that particular wordpress install’s root?
http://www.website-name/demos/
Ryan Sechrest
January 13, 2014 at 2:38 pm
Bill, I revised the function to be a little more flexible and it should also now work with WordPress installed in a subdirectory. Take another look at the code in step 1.
Bill
January 18, 2014 at 7:02 pm
Thanks for the reply Ryan. I must be doing something wrong, because I cant get it to work. First, in step 1, you forgot the wordcustom
in the function. Line 8 should be:function custom_post_type_link($permalink, $post, $leavename) {
But regardless, I tried this but it didnt work. Not sure if this matters or not, but the site is located in this pathpublic_html/SUBFOLDER/the-wordpress-install-is-here
The custom post type name is “neighborhoods”. Here is my code:
/* Remove the neighborhoods slug from published post permalinks. Only affect our CPT though*/
add_filter(
'post_type_link',
'custom_post_type_link',
10,
3
);
function custom_post_type_link($permalink, $post, $leavename) {
if(is_object($post) && property_exists($post, 'post_type')) {
switch($post->post_type) {
case 'neighborhoods':
$permalink = str_replace('/' . $post->post_type, '', $permalink);
break;
}
}
return $permalink;
}
/*
* Some hackery to have WordPress match postname to any of our public post types
* All of our public post types can have /post-name/ as the slug, so they better be unique across all posts
* Typically core only accounts for posts and pages where the slug is /post-name/
*/
add_action(
'pre_get_posts',
'custom_pre_get_posts'
);
function custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
$post_name = $query->get('pagename');
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
switch($post_type) {
case 'neighborhoods':
$query->set('neighborhoods', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
return $query;
}
Ryan Sechrest
January 19, 2014 at 5:36 am
You’re right, I didn’t test my quick update and it contained an error. Please take another look, this time tested, and it should now work for you.
Bill
January 19, 2014 at 6:43 am
Hi Ryan…
Ok, I tried new code …the permalink does change int he editor screen …the slug is not there, but I get the 404. I tried resetting permalinks just to make sure there wasn’t an issue there with the 404, but still no dice.
I will paste what I have below for the CPT just in case it is something on my end…
/** Remove slug from custom post type for "neighborhoods" custom post ype */
add_filter(
'post_type_link',
'custom_post_type_link',
10,
3
);
function custom_post_type_link($permalink, $post, $leavename) {
if (!gettype($post) == 'post') {
return $permalink;
}
switch ($post->post_type) {
case 'neighborhoods':
$permalink = get_home_url() . '/' . $post->post_name . '/';
break;
}
return $permalink;
}
/*
* Some hackery to have WordPress match postname to any of our public post types
* All of our public post types can have /post-name/ as the slug, so they better be unique across all posts
* Typically core only accounts for posts and pages where the slug is /post-name/
*/
add_action(
'pre_get_posts',
'custom_pre_get_posts'
);
function custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
$post_name = $query->get('pagename');
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
switch($post_type) {
case 'neighborhoods':
$query->set('neighborhoods', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
return $query;
}
/*****nd this is my custom post type:******/
/* Add Custom Post Type for Neighborhoods*/
add_action( 'init', 'create_my_post_types2', 2 );
function create_my_post_types2() {
register_post_type( 'neighborhoods',
array(
'labels' => array(
'name' => __( 'Neighborhoods' ),
'singular_name' => __( 'neighborhoods' ),
'add_new' => __( 'Add New' ),
'add_new_item' => __( 'Add neighborhood' ),
'edit' => __( 'Edit' ),
'edit_item' => __( 'Edit neighborhood' ),
'new_item' => __( 'New neighborhood' ),
'view' => __( 'View neighborhood' ),
'view_item' => __( 'View neighborhood' ),
'search_items' => __( 'Search neighborhoods' ),
'not_found' => __( 'No neighborhoods found' ),
'not_found_in_trash' => __( 'No neighborhoods found in Trash' ),
'parent' => __( 'Parent neighborhoods' ),
),
'public' => true,
'show_ui' => true,
'publicly_queryable' => true,
'exclude_from_search' => false,
'menu_position' => 20,
'menu_icon' => get_stylesheet_directory_uri() . '/images/neighborhood-icon.png',
'hierarchical' => false,
'query_var' => false,
'supports' => array( 'title', 'editor', 'excerpt', 'custom-fields', 'thumbnail', 'page-attributes', 'author', 'comments' ,'genesis-layouts', 'genesis-seo' , 'trackbacks', 'revisions' ),
'rewrite' => array( 'slug' => 'neighborhoods', 'with_front' => false ),
'taxonomies' => array( 'post_tag', 'category'),
'has_archive' => true,
'can_export' => true,
)
);
}
Ryan Sechrest
January 19, 2014 at 6:34 pm
Here is the code I used for testing https://gist.github.com/ryansechrest/03b3caa9f12c2d70f7bc. Throw this code inwp-content/mu-plugins/remove-cpt-slug.php
and create a parent and childservice
post. Let me know if you have the same issues as with yourneighborhoods
post type.
bill
January 21, 2014 at 8:05 am
thanks Ryan. I followed your instructions…I still get a 404 for both service posts I create (one parent and one child)
Ryan Sechrest
January 21, 2014 at 12:24 pm
Maybe you have a different kind of WordPress configuration or a plugin that is preventing this from working? I downloaded WordPress 3.8, installed it in a subdirectory as a single site, and then only installed that plugin I referenced above, and that worked for me.
As a side note, this is also working on a production site running multisite, where WordPress is in a subdirectory, but served from the root.
The ultimate test would be to setup a clean WordPress, just to rule out a configuration/setup/plugin issue. If that works, you know it has something to do with your sandbox.
With regard to debugging the sandbox, since the problem seems to be related to step #2 (since the URL seems to be accurate), I would start with printing out various variables. Something like:
add_action(
'pre_get_posts',
'custom_pre_get_posts'
);
function custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
echo '$query before: <pre>' . print_r($query, true) . '</pre>';
$post_name = $query->get('pagename');
echo '$post_name: <pre>' . $post_name . '</pre>';
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
echo '$post_type: <pre>' . $post_type . '</pre>';
switch($post_type) {
case 'services':
$query->set('services', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
echo '$query after: <pre>' . print_r($query, true) . '</pre>';
return $query;
}
Bill
January 21, 2014 at 1:05 pm
Thanks for your help Ryan!
….I deactivated all plugins, and switched to 2014 theme…. still same issue. 404 error.
Here is my set up: I originally had a website in the main public_html folder. Then over the years, I added three add-on domains for this hostgator account. so it was set up like this:
public_html/MAIN WORDPRESS INSTALL/ (then I have three different folders here….one for each of the three add-on websites)
Each of the three folders has its own WordPress install. Then a year or so ago, I deleted the main wordpress install from the server because I dont use that site anymore…. but I kept the three ass-on websites….. so it looks like this
public_html/website1.com or
public_html/website2.com or
public_html/website2.com
It’s the samepublic_html/
but with three different add-on domains, and the original wordpress install is not there anymore….just the addon folders. Not sure why this would make a difference, but maybe it does?
Ryan Sechrest
January 21, 2014 at 1:33 pm
The individual WordPress sites (using add-on domains) should be isolated from each other, so I don’t think that’s the problem.
Re: WordPress theme and plugin deactivation, while that is a good test, there could still be some configuration in the database that could prevent this from working. A better test would be a fresh install with a newly setup database.
Also, have you checked the output of the variables mentioned in my previous comment?
Daniel
January 30, 2014 at 9:21 pm
Hi Ryan,
Nice job. However I hope you can help.
I’m wondering what if you want to replace the post type slug with my taxonomy term slug. How do I do that?
Cheers.
Ryan Sechrest
January 30, 2014 at 10:49 pm
You could try something like this. It assumes you are using the post_tag
taxonomy and only have one term. If you have more than one term, it will use the first one. In addition, while the permalink will generate based on your selected term, it doesn’t validate the term when parsing the URL, meaning the page would render with any term, really.
function post_type_link($permalink, $post, $leavename) {
if (!gettype($post) == 'post') {
return $permalink;
}
switch ($post->post_type) {
case 'service':
$permalink = get_home_url() . '/';
$terms = wp_get_post_terms($post->ID, 'post_tag', array('number' => 1));
if (is_array($terms) && isset($terms[0]) && property_exists($terms[0], 'slug')) {
$permalink .= $terms[0]->slug . '/';
}
$permalink .= $post->post_name . '/';
break;
}
return $permalink;
}
function pre_get_posts($query) {
global $wpdb;
if (!$query->is_main_query()) {
return;
}
$post_name = $query->get('pagename');
$last_pos = strrpos($post_name, '/');
if ($last_pos !== false) {
$post_name = substr($post_name, $last_pos + 1);
}
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
switch ($post_type) {
case 'service':
if (substr_count($query->get('pagename'), '/') != 1) {
return $query;
}
$query->set('service', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
return $query;
}
Yumna
March 11, 2014 at 12:43 am
Hi Ryan,
I am trying to change my cpt urls using your code. I could remove slug from parent post urls, but it fails on child post urls. My cpt is hierarchical. I modified the post_type_link hooked function as below and now it changes link for child pages as well
function custom_post_type_link($permalink, $post, $leavename) {
if ((!gettype($post) == 'post') ){
return $permalink;
}
switch ($post->post_type) {
case 'user-page':
//var_dump($post);
if($post->post_parent!=0){
$permalink = get_home_url() . '/' . get_post($post->post_parent)->post_title. '/'. $post->post_name ;
}else{
$permalink = get_home_url() . '/' . $post->post_name . '/';
}
break;
}
return $permalink;
}
Now the permalinks are created correctly, but the problem is that i get a 404 on child posts. I have dumped the query on both parent and child posts and i have noticed that on child post, the query_var attachment is set and no other query_vars are set. Please help if possible. Thanks.
Ryan Sechrest
March 12, 2014 at 9:47 am
OK, first you’ll want to changepost_title
topost_name
on line 10, because otherwise you might have space and capitalization issues in the URL. I’d also add the trailing slash.
$permalink = get_home_url() . '/' . get_post($post->post_parent)->post_name . '/' . $post->post_name . '/';
Second, and this is the main problem, the post name being used to find your child page in the database contains the parent slug, in other words,parent-page/child-page
, but there is no post with a post name like that, hence the page not found error.
That means you have to strip out the parent slug or simply take the contents after the last slash.
If you add the following after$post_name = $query->get('pagename');
in thepre_get_posts
hook, it should work:
$last_pos = strrpos($post_name, '/');
if ($last_pos !== false) {
$post_name = substr($post_name, $last_pos + 1);
}
Let me know if this works.
Irvin
April 29, 2014 at 2:50 am
Hi Ryan,
I may need help on this: http://pastebin.com/Qwaf8wuD
The Parent works well actually but the Child pages are giving me 404:
domain.com/parent1/weather
domain.com/parent2/weather
domain.com/parent2/weather
anything did I miss?
Ryan Sechrest
April 29, 2014 at 9:12 am
I don’t think you followed my tutorial very closely, because your code looks completely different. At first glance, though, I believe you’re coming up empty here:
if ( ! empty( $query->query['name'] ) ) {
$query->set( 'post_type', array( 'post', 'resort', 'page' ) );
}
Try swapping$query->query['name']
with$query->get['pagename']
.
Yana
January 13, 2015 at 6:51 am
Hello Ryan,
thank you for this great post, it was exactly what I am looking for.
Just one question regarding to Tomas post if this function or plugin will work with WPML:
I use your function for a site which is published in two languages (German source and English using WPML plugin). I have different custom post types (on of them is members, not hierarchical) which are first filled in an overview page (simple loop) including an link to the detail page of the member. The overview works as expected but when I try to get the detail page it only works in the German version, the English version throws an error 404. I would really appreciate any advise which will point me to the right direction.
Thank you,
Yana
Ryan Sechrest
January 13, 2015 at 9:55 am
Looking back at Tomas’ post, I didn’t even realize that he was talking about WPML the plugin and not WPMU… I skipped right over that!
"I use your function for a site which is published in two languages (German source and English using WPML plugin)."
So your main site isexample.org
and your translated site is something likeexample.org/?lang=en
, right?
"I have different custom post types (on of them is members, not hierarchical)"
So member pages look something like this, right?
*example.org/members/john-doe
*example.org/members/jane-doe
*example.org/members/foo-bar
"which are first filled in an overview page (simple loop) including an link to the detail page of the member"
When you say overview and detail page, do you mean something like:
Overview:example.org/members/john-doe
— Contains picture, name, basic info.
Detail:example.org/members/john-doe/details
— Contains expanded profile, posts published, etc.
"The overview works as expected but when I try to get the detail page it only works in the German version, the English version throws an error 404."
So, this works:
*example.org/members/john-doe
*example.org/members/john-doe/?lang=en
*example.org/members/john-doe/details
But this does not:
*example.org/members/john-doe/details/?lang=en
Did I understand the problem correctly?
Yana
January 13, 2015 at 2:04 pm
Hello Ryan,
thank you for your response.
"ple.org/?lang=en, right?"
No it isexample.org/en
and for german (source language) onlyexample.org/
My german overview page has this url format:myurl.com/my-page/my-subpage/sub-sub-page/
with a list of all members.
It is a loop through the cpt (in the template file) with listing the members in this page showing their picture and small informations, including a link to their detail page.
The english version has this url:myurl.com/en/my-page-en/my-subpage-en/sub-sub-page-en/
What I do is using your function to remove the slug from cpt an add a new path to get the following result for the detail page in the url (german):
myurl.com/my-page/my-subpage/sub-sub-page/john-doe
and for the english version:
myurl.com/en/my-page-en/my-subpage-en/sub-sub-page-en/john-doe
I have an if statement in the function to get the current language code and use to two different path for the german and english version.
Everything works perfect in the german version but when I try to call the detail page of e.g.myurl.com/my-page/my-subpage/sub-sub-page-en/john-doe
I get the error 404.
Thank for your help and if you need more information please let me know.
Yana
Ryan Sechrest
January 13, 2015 at 2:52 pm
You haveen
several times in the URL, but that’s just to indicate that it is an English slug, right? The only thing used to determine language isen
right after the domain, correct?
In other words, this works:
myurl.com/seite/sub-seite/sub-sub-seite/john-doe
But this does not work:
myurl.com/en/page/sub-page/sub-sub-page/john-doe
Did I get that right?
If so, it seems part 1, building the URL, is working fine for you, but you’re having trouble with part 2, which is rebuilding the query to then display the resulting data.
Yana
January 14, 2015 at 4:47 am
Hello Ryan,
yes, exactly.
This is working
myurl.com/seite/sub-seite/sub-sub-seite/john-doe
This is not working
myurl.com/en/page/sub-page/sub-sub-page/john-doe
Thank you
Yana
Ryan Sechrest
January 14, 2015 at 9:05 am
OK, can you post the code you’re using for part 2 with the modifications you made?
Yana
January 14, 2015 at 9:33 am
Here is the code I am using:
add_filter('post_type_link', 'svr_custom_post_type_link', 10, 3);
function svr_custom_post_type_link($permalink, $post, $leavename) {
if (!gettype($post) == 'post') {
return $permalink;
}
switch ($post->post_type) {
case 'mitglieder-svr':
if (ICL_LANGUAGE_CODE == 'de') {
$permalink = home_url( '/' ) . 'sachverstaendigenrat/wir-ueber-uns/mitglieder-svr/' . $post->post_name . '/';
}
if (ICL_LANGUAGE_CODE == 'en') {
$permalink = home_url( '/' ) . 'expert-council/about-us/council-members/' . $post->post_name . '/';
}
break;
case 'mitarbeiter':
if (ICL_LANGUAGE_CODE == 'de') {
$permalink = home_url( '/' ) . 'sachverstaendigenrat/wir-ueber-uns/geschaeftsstelle/' . $post->post_name . '/';
}
if (ICL_LANGUAGE_CODE == 'en') {
$permalink = home_url( '/' ) . 'expert-council/about-us/berlin-office/' . $post->post_name . '/';
}
break;
}
return $permalink;
}
And the pre_get_post
functions look like this:
add_action('pre_get_posts', 'svr_custom_pre_get_posts');
function svr_custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
$post_name = $query->get('name');
$result = $wpdb->get_row(
$wpdb->prepare(
'SELECT wpp1.post_type, wpp2.post_name AS parent_post_name FROM ' . $wpdb->posts . ' as wpp1 LEFT JOIN ' . $wpdb->posts . ' as wpp2 ON wpp1.post_parent = wpp2.ID WHERE wpp1.post_name = %s LIMIT 1',
$post_name
)
);
if(!empty($result->post_type)) {
switch($result->post_type) {
case 'mitglieder-svr':
if ($result->parent_post_name !== '') {
$post_name = $result->parent_post_name . '/' . $post_name;
}
$query->set('mitglieder-svr', $post_name);
$query->set('post_type', $result->post_type);
$query->is_single = true;
$query->is_page = false;
break;
case 'mitarbeiter':
if ($result->parent_post_name !== '') {
$post_name = $result->parent_post_name . '/' . $post_name;
}
$query->set('mitarbeiter', $post_name);
$query->set('post_type', $result->post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
}
return $query;
}
It seems that the first function causes also an error in the backend, all page, post and cpt linstings disappeared this morning. By removing the function everthing works again.
Ryan Sechrest
January 14, 2015 at 2:21 pm
@Yana
Can you print out$result
on a German and an English error page? You can do that with something like:
echo '<pre>' . print_r($result, true) . '</pre>';
"It seems that the first function causes also an error in the backend, all page, post and cpt linstings disappeared this morning. By removing the function everthing works again."
Do you know what the error was?
Yana
January 15, 2015 at 8:05 am
I was wrong, it is not the first function, it is thepre_get_post
function which causes the problem. After cache clearing the whole site throws an 404 error. It seems that in my case the first cptmitglieder-svr
is allways used in every query.
stdClass Object
(
[post_type] => mitglieder-svr
[parent_post_name] =>
)
Ryan Sechrest
January 16, 2015 at 9:00 am
Give the original code a try for pre_get_posts
(especially the query part that gets the post type), since you are not exactly doing what I’m doing in the blog post (which is writing all permalinks to the root without the post type slug).
Trieu To
February 2, 2015 at 6:46 am
Hi Ryan
I use your code but at step 2 it not working.
This is my code:
function custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
$post_name = $query->get('pagename');
$result = $wpdb->get_row(
$wpdb->prepare(
'SELECT wpp1.post_type, wpp2.post_name AS parent_post_name FROM ' . $wpdb->posts . ' as wpp1 LEFT JOIN ' . $wpdb->posts . ' as wpp2 ON wpp1.post_parent = wpp2.ID WHERE wpp1.post_name = %s LIMIT 1',
$post_name
)
);
if(!empty($result->post_type)) {
switch($result->post_type) {
case 'chapter':
if ($result->parent_post_name !== '') {
$post_name = $result->parent_post_name . '/' . $post_name;
}
$query->set('chapter', $post_name);
$query->set('post_type', $result->post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
}
return $query;
}
Trieu To
February 2, 2015 at 8:01 am
Dear Ryan
I want to few character to url example:
add_filter(
'post_type_link',
'custom_post_type_link',
10,
3
);
function custom_post_type_link($permalink, $post, $leavename) {
if (!gettype($post) == 'post') {
return $permalink;
}
switch ($post->post_type) {
case 'services':
$permalink = get_home_url() . '/' .'example'. $post->post_name . '/';
break;
}
return $permalink;
}
But step 2 not work. How can i fix it? and How can i add any character to my custom post type slug?
Ryan Sechrest
February 3, 2015 at 9:01 am
Let’s say your post name is “foobar” and let’s say you build a URL as follows:
example.org/examplefoobar
When the query is performed to look up the post, there is no post in your database called “examplefoobar,” which is why step 2 is not working.
If you are adding “example” in the post name, you must remove it again prior to making the query. So, on line 8 in your first post, you could try something like this:
$post_name = str_replace('example', '', $query->get('pagename'));
Note that in this case, regardless of where “example” occurs in the string, it will be removed, so depending on your rules, you may want to refine it.
Better yet, though, if you changed it toexample/foobar
instead ofexamplefoobar
, that might be a little bit more reliable, as you could just grab everything after the last slash, but at that point, you might as well just change the slug of the post type toexample
, because then you don’t need any of this extra code.
You can find this argument in therewrite
section on the register_post_type page in the WordPress codex.
$args = array(
'rewrite' => array(
'slug' => 'example'
)
);
register_post_type('something', $args);
Teilmann
February 16, 2015 at 2:24 am
Hello Ryan,
I have implemented your solution on my local environment, and it works like a charm. However, when i do the same on the online solution, some links break, and i cannot save stuff in the administration panel. (posts, permalinks and more). Any thoughts?
Teilmann
February 16, 2015 at 3:00 am
Nevermind Ryan, i seemed to fix the problem. The former programmer on the site im working on, made a bunch of whitespaces in the online version of the functions.php file, which caused the white screen of death.
thanks for the awesome solution
Ryan Sechrest
February 16, 2015 at 9:56 am
Good deal– glad it’s working for you!
Thomas Teilmann
February 17, 2015 at 2:13 am
Hello again, it seems i have found yet another problem :/
When i implemented your solution, i changed the permalinks to some of my custom post types, so that they linked to the root of the website. By doing that, i now have more than one link to my custom post types. The links i had before i changed it, is still active, and thats not good according to google (SEO), because the pages are interpreted as duplicates (obviously).
Any idea how to fix this?
Pierre
May 28, 2015 at 4:48 am
Hi ryan, Trying to use your solution to remove “product” from slug in woocommerce
But the query in get is quite empty (at least for name and pagename) , and i got 404.
'name' => '',
'static' => '',
'pagename' => '',
This is the code, in case you spot any issue…
khubbaib
August 29, 2015 at 3:17 pm
You are rock ryan… Its help me alot,,, In first try it was not working but now it working… Thank you so much…
Colir
November 12, 2015 at 9:57 am
Hi.
DO you think is possible to just remove the cpt name from a slug, but keep the hierachy url ?
ex: http://www.domain.com/cpt-name/parent-post/child-post
to http://www.domain.com/parent-post/child-post
Your code work well, but as you need, also remove the hierachy
thanks
Colir
November 13, 2015 at 7:06 am
Hi. Thanks for this solution.
However, i can’t get a solution to keep the parent post name in the url
ex: http://www.domain.com/cpt-name/cpt-parent-page/cpt-child-page
to : http://www.domain.com/cpt-parent-page/cpt-child-page
any idea ?
thanks a lot
Ryan Sechrest
November 13, 2015 at 12:30 pm
Hi Colir,
Take a look at this Gist I put together– it should do the trick.
colir
November 16, 2015 at 4:24 am
i think i‘ve found a first probleme $query->get(‘pagename’);
return an empty string…
Colir
November 16, 2015 at 4:10 am
Thnaks a lot for your answer.
The url is good: http://www.domain.com/parent_cpt_page_name/child_cpt_page_name
However i have a 404 error on my child page.
I create my CPT with the CPT UI plugin (so i remove your cpt definition in your plugin)
my CPT name is ‘galerie’ so i also remove all ‘service’ string by ‘galerie’ in you plugin.
Colir
November 16, 2015 at 7:36 am
Yo Ryan
i’ve spent time on the problem and here is what happen :
– i can’t get the post type in thepre_get_posts
function because after apply thepost_type_link
filter,$post_name = $page_name = $query->get('pagename');
is empty.
So here is what i’m done. this work but it’s really tricky
function remove_cpt_slug( $permalink, $post, $leavename ) {
if ( 'galerie' != $post->post_type || 'publish' != $post->post_status ) {
return $post_link;
}
$permalink = str_replace($post->post_type . '/', '', $permalink);
return $permalink;
}
add_filter( 'post_type_link', 'remove_cpt_slug', 10, 3 );
function pre_get_posts( $query ) {
global $wp_query;
if ( ! $query->is_main_query())
return;
$post_name = $page_name = $query->get('pagename');
$page_id = $query->get('page_id');
if ( empty( $page_name ) && $page_id == 0) {
$query->set( 'post_type', 'galerie' );
$query->is_single = true;
$query->is_page = false;
}
}
add_action( 'pre_get_posts', 'pre_get_posts' );
note: i also test the$page_id
, because my home page is a custom template page.
on this page (home), the$post_name
is also empty, but not his ID.
How to you think we can improve the things ?
thanks
Ryan Sechrest
November 16, 2015 at 9:02 am
Hi Colir,
Print out$query
inpre_get_posts
using something like:
wp_die('<pre>'.print_r($query, true).'</pre>');
And let me know what the result is.
You need to obtain the post type, otherwise your hook will fire on every request and for all post types, which will most likely break other aspects of WordPress.
Also, just to confirm, are you running the latest version of WordPress? I tested this with version 4.3.1 and a non-multisite install.
Colir
November 17, 2015 at 7:18 am
Here is the result.
For info, my child post name is CEP.
As you can see the query let appear it as an attachment, but i didn’t know why (this is not the case if didnt filter the permalink).
My WP version is 4.3.1
WP_Query Object
(
[query_vars] => Array
(
[attachment] => cep
[error] =>
[m] =>
[p] => 0
[post_parent] =>
[subpost] =>
[subpost_id] =>
[attachment_id] => 0
[name] =>
[static] =>
[pagename] =>
[page_id] => 0
[second] =>
[minute] =>
[hour] =>
[day] => 0
[monthnum] => 0
[year] => 0
[w] => 0
[category_name] =>
[tag] =>
[cat] =>
[tag_id] =>
[author] =>
[author_name] =>
[feed] =>
[tb] =>
[paged] => 0
[comments_popup] =>
[meta_key] =>
[meta_value] =>
[preview] =>
[s] =>
[sentence] =>
[fields] =>
[menu_order] =>
[category__in] => Array
(
)
[category__not_in] => Array
(
)
[category__and] => Array
(
)
[post__in] => Array
(
)
[post__not_in] => Array
(
)
[tag__in] => Array
(
)
[tag__not_in] => Array
(
)
[tag__and] => Array
(
)
[tag_slug__in] => Array
(
)
[tag_slug__and] => Array
(
)
[post_parent__in] => Array
(
)
[post_parent__not_in] => Array
(
)
[author__in] => Array
(
)
[author__not_in] => Array
(
)
)
[tax_query] =>
[meta_query] =>
[date_query] =>
[post_count] => 0
[current_post] => -1
[in_the_loop] =>
[comment_count] => 0
[current_comment] => -1
[found_posts] => 0
[max_num_pages] => 0
[max_num_comment_pages] => 0
[is_single] => 1
[is_preview] =>
[is_page] =>
[is_archive] =>
[is_date] =>
[is_year] =>
[is_month] =>
[is_day] =>
[is_time] =>
[is_author] =>
[is_category] =>
[is_tag] =>
[is_tax] =>
[is_search] =>
[is_feed] =>
[is_comment_feed] =>
[is_trackback] =>
[is_home] =>
[is_404] =>
[is_comments_popup] =>
[is_paged] =>
[is_admin] =>
[is_attachment] => 1
[is_singular] => 1
[is_robots] =>
[is_posts_page] =>
[is_post_type_archive] =>
[query_vars_hash:WP_Query:private] => d5501ed18d237da795d238464d9f7e78
[query_vars_changed:WP_Query:private] =>
[thumbnails_cached] =>
[stopwords:WP_Query:private] =>
[compat_fields:WP_Query:private] => Array
(
[0] => query_vars_hash
[1] => query_vars_changed
)
[compat_methods:WP_Query:private] => Array
(
[0] => init_query_flags
[1] => parse_tax_query
)
[query] => Array
(
[attachment] => cep
)
)
Ryan Sechrest
November 18, 2015 at 1:22 am
Hi Colir,
I see the attachment, and that doesn’t appear to be normal. My guess is that either your theme or a plugin is responsible for that. It’s difficult to troubleshoot without having more context. I ran my code in a brand new WordPress installation, so I know there was nothing interfering there.
That said, I would test the code in a brand new WordPress installation and then add your theme and plugins back, one by one, to isolate what might be causing this.
Colir
November 18, 2015 at 9:11 am
Ok. Thanks a lot for your help.
I will try to setup a news WP and see
Colir
November 18, 2015 at 9:25 am
Ryan, i‘ve just setup a new clean install of the last version of WP,
and active only one plugin ‘Custom Post Type UI’.
I’ve setup a new cpt with the hierachie param on.
I’ve the same result as you can see
WP_Query Object
(
[query_vars] => Array
(
[attachment] => child-galerie
[error] =>
[m] =>
[p] => 0
[post_parent] =>
[subpost] =>
[subpost_id] =>
[attachment_id] => 0
[name] =>
[static] =>
[pagename] =>
[page_id] => 0
[second] =>
[minute] =>
[hour] =>
[day] => 0
[monthnum] => 0
[year] => 0
[w] => 0
[category_name] =>
[tag] =>
[cat] =>
[tag_id] =>
[author] =>
[author_name] =>
[feed] =>
[tb] =>
[paged] => 0
[comments_popup] =>
[meta_key] =>
[meta_value] =>
[preview] =>
[s] =>
[sentence] =>
[fields] =>
[menu_order] =>
[category__in] => Array
(
)
[category__not_in] => Array
(
)
[category__and] => Array
(
)
[post__in] => Array
(
)
[post__not_in] => Array
(
)
[tag__in] => Array
(
)
[tag__not_in] => Array
(
)
[tag__and] => Array
(
)
[tag_slug__in] => Array
(
)
[tag_slug__and] => Array
(
)
[post_parent__in] => Array
(
)
[post_parent__not_in] => Array
(
)
[author__in] => Array
(
)
[author__not_in] => Array
(
)
)
[tax_query] =>
[meta_query] =>
[date_query] =>
[post_count] => 0
[current_post] => -1
[in_the_loop] =>
[comment_count] => 0
[current_comment] => -1
[found_posts] => 0
[max_num_pages] => 0
[max_num_comment_pages] => 0
[is_single] => 1
[is_preview] =>
[is_page] =>
[is_archive] =>
[is_date] =>
[is_year] =>
[is_month] =>
[is_day] =>
[is_time] =>
[is_author] =>
[is_category] =>
[is_tag] =>
[is_tax] =>
[is_search] =>
[is_feed] =>
[is_comment_feed] =>
[is_trackback] =>
[is_home] =>
[is_404] =>
[is_comments_popup] =>
[is_paged] =>
[is_admin] =>
[is_attachment] => 1
[is_singular] => 1
[is_robots] =>
[is_posts_page] =>
[is_post_type_archive] =>
[query_vars_hash:WP_Query:private] => fad285f1d17b209d7e24097fedf24639
[query_vars_changed:WP_Query:private] =>
[thumbnails_cached] =>
[stopwords:WP_Query:private] =>
[compat_fields:WP_Query:private] => Array
(
[0] => query_vars_hash
[1] => query_vars_changed
)
[compat_methods:WP_Query:private] => Array
(
[0] => init_query_flags
[1] => parse_tax_query
)
[query] => Array
(
[attachment] => child-galerie
)
)
Colir
November 18, 2015 at 9:38 am
I’ve made a new test :
removing custom Post Type UI and put directly your gist…same effect…
The cptservice
is seen as an attachment
Ryan Sechrest
November 20, 2015 at 12:07 am
Hi Colir,
Can you confirm where you placed the statement in the code and which page you were trying to load when it printed that out?
Colir
November 20, 2015 at 7:20 am
I place it in the “pre_get_post” and i try to load
a child page of my cpt
function pre_get_posts($query) {
global $wpdb;
wp_die('<pre>'.print_r($query, true).'</pre>');
// If current query is WordPress' main query
if (!$query->is_main_query()) {
return;
}
// Get page name from query
$post_name = $page_name = $query->get('pagename');
// Determine if post name contains slash
$last_pos = strrpos($post_name, '/');
// If post name contains slash, it's hierarchical
if ($last_pos !== false) {
// Extract actual post name
$post_name = substr($post_name, $last_pos + 1);
}
// Retrieve post type based on post name
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
// Only process specified post types
switch ($post_type) {
case 'service':
$query->set('service', $page_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
return $query;
}
Ryan Sechrest
November 20, 2015 at 8:27 am
Hi Colir,
Try putting it right before:
return $query;
Also, when I was testing, I was using the Twenty Fifteen theme– which one were you using?
Colir
November 24, 2015 at 1:39 am
Hi Ryan.
First at all thanks a lot for the timing you spending to help me.
So it’s really stange.
I’m also woth the twenty Fifteen theme.
After putting the die just before $query, this work well.
So i ‘ve donwload Custom Post Type UI, set another type and change the Git.
After this done, any thing work as except.
I’ve got an 404 on my custom type
And for the cpt “Services” (which work well before), i’m falling with the ‘attachment problem’ even if i remove the CPT plugin…
sorry for my poor english i’m french.
thanks a lot
Colir
November 24, 2015 at 1:45 am
Here the modified git i use
__('Services', PS),
'singular_name' => __('Service', PS),
'menu_name' => __('Services', PS),
'all_items' => __('All Services', PS),
'add_new' => __('Add Service', PS),
'add_new_item' => __('Add New Service', PS),
'edit_item' => __('Edit Service', PS),
'new_item' => __('New Service', PS),
'view_item' => __('View Service', PS),
'search_items' => __('Search Services', PS),
'not_found' => __('No services found', PS),
'not_found_in_trash' => __('No services found in trash', PS),
'parent_item_colon' => __('Parent Service', PS)
);
$description = __('Services', PS);
$supports = array(
'title',
'editor',
'page-attributes'
);
$taxonomies = array();
$arguments = array(
'label' => $label,
'labels' => $labels,
'description' => $description,
'public' => true,
'show_ui' => true,
'menu_position' => 20,
'hierarchical' => true,
'supports' => $supports,
'taxonomies' => $taxonomies,
'has_archive' => false,
'rewrite' => true,
'query_var' => true,
'can_export' => false
);
register_post_type('service', $arguments);
}
function register_post_type_galerie() {
$label = __('Galerie', PS);
$labels = array(
'name' => __('Galeries', PS),
'singular_name' => __('Galerie', PS),
'menu_name' => __('Galeries', PS),
'all_items' => __('All Galeries', PS),
'add_new' => __('Add Galerie', PS),
'add_new_item' => __('Add New Galerie', PS),
'edit_item' => __('Edit Galerie', PS),
'new_item' => __('New Galerie', PS),
'view_item' => __('View Galerie', PS),
'search_items' => __('Search Galeries', PS),
'not_found' => __('No services found', PS),
'not_found_in_trash' => __('No services found in trash', PS),
'parent_item_colon' => __('Parent Galerie', PS)
);
$description = __('Galeries', PS);
$supports = array(
'title',
'editor',
'page-attributes'
);
$taxonomies = array();
$arguments = array(
'label' => $label,
'labels' => $labels,
'description' => $description,
'public' => true,
'show_ui' => true,
'menu_position' => 20,
'hierarchical' => true,
'supports' => $supports,
'taxonomies' => $taxonomies,
'has_archive' => false,
'rewrite' => true,
'query_var' => true,
'can_export' => false
);
register_post_type('galerie', $arguments);
}
/******************************************************************************/
function hook_post_type_link() {
add_filter(
'post_type_link',
NS . 'post_type_link',
10,
3
);
}
function post_type_link($permalink, $post, $leavename) {
// Remove post type slug from these post types
$post_types = array('service','galerie');
// If current post doesn't have post type listed above
if(!in_array($post->post_type, $post_types)) {
return $permalink;
}
// Remove post type slug from permalink
$permalink = str_replace($post->post_type . '/', '', $permalink);
return $permalink;
}
/*************************************************************************/
function hook_pre_get_posts() {
add_action(
'pre_get_posts',
NS . 'pre_get_posts'
);
}
function pre_get_posts($query) {
global $wpdb;
// If current query is WordPress' main query
if (!$query->is_main_query()) {
return;
}
// Get page name from query
$post_name = $page_name = $query->get('pagename');
// Determine if post name contains slash
$last_pos = strrpos($post_name, '/');
// If post name contains slash, it's hierarchical
if ($last_pos !== false) {
// Extract actual post name
$post_name = substr($post_name, $last_pos + 1);
}
// Retrieve post type based on post name
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
// Only process specified post types
switch ($post_type) {
case 'service':
$query->set('service', $page_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
case 'galerie':
$query->set('galerie', $page_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
wp_die('<pre>'.print_r($query, true).'</pre>');
return $query;
}
Ash Bryant
April 6, 2016 at 7:15 am
Hi Ryan,
I’ve been trying to get this to work and in hope to find a solution, I also came across this before yours ( http://kellenmace.com/remove-custom-post-type-slug-from-permalinks/ ) which works, but not on a multisite install it would seem ( just on the main site ), so I continued to look for help and came here. I saw that you said your solution should work on a multisite install, so I thought ‘great!’, but I’ve been trying to get this to work for a little while now, and keep getting a 404 error.
I have a series of cpt’s that I want to do this with ( ‘excursion’, ‘hotel’, ‘offer’, ‘tour’ ), I have these setup on a multisite install of WP using subdomains. I have also got domain mapping on them, but I have tried your solution first without this active, but with no joy.
Can you help?
CODE
add_filter(
'post_type_link',
'custom_post_type_link',
10,
3
);
function custom_post_type_link($permalink, $post, $leavename) {
if (!gettype($post) == 'post') {
return $permalink;
}
switch ($post->post_type) {
case 'excursion':
$permalink = get_home_url() . '/' . $post->post_name . '/';
break;
case 'hotel':
$permalink = get_home_url() . '/' . $post->post_name . '/';
break;
case 'offer':
$permalink = get_home_url() . '/' . $post->post_name . '/';
break;
case 'tour':
$permalink = get_home_url() . '/' . $post->post_name . '/';
break;
}
return $permalink;
}
add_action(
'pre_get_posts',
'custom_pre_get_posts'
);
function custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
echo '$query before: <pre>' . print_r($query, true) . '</pre>';
$post_name = $query->get('pagename');
echo '$post_name: <pre>' . $post_name . '</pre>';
$result = $wpdb->get_row(
$wpdb->prepare(
'SELECT wpp1.post_type, wpp2.post_name AS parent_post_name FROM ' . $wpdb->posts . ' as wpp1 LEFT JOIN ' . $wpdb->posts . ' as wpp2 ON wpp1.post_parent = wpp2.ID WHERE wpp1.post_name = %s LIMIT 1',
$post_name
)
);
switch($post_type) {
case 'excursion':
$query->set('excursion', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
case 'hotel':
/*
if ($result->parent_post_name !== '') {
$post_name = $result->parent_post_name . '/' . $post_name;
}
*/
$query->set('hotel', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
case 'offer':
$query->set('offer', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
case 'tour':
$query->set('tour', $post_name);
$query->set('post_type', $post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
echo '$query after: <pre>' . print_r($query, true) . '</pre>';
}
Ryan Sechrest
April 6, 2016 at 9:29 am
Hi Ash,
Does my code work for you on the first site in a multisite environment and just not on the other sites, or does it not work at all?
Ash Bryant
April 7, 2016 at 2:55 am
Hi Ryan,
It doesn’t seem to work at all. On the main site thecustom_post_type_link
function seems to work as the url has those parts removed. But thecustom_pre_get_posts
function doesn’t seem to work as I get a 404.
On the other sites of the install it doesn’t do anything at all, the pages load without removing the parts and therefore load as ‘normal’.
Ryan Sechrest
April 7, 2016 at 10:30 am
Just to be sure, can you visit the Permalinks page to flush your rewrite rules and then see if you still get a 404?
Ash Bryant
April 8, 2016 at 7:30 am
Yep already done that :/
Ash Bryant
April 8, 2016 at 8:58 am
If it helps these are my CPT & Taxonomies
add_action( 'init', 'cptui_register_my_cpts' );
function cptui_register_my_cpts() {
$labels = array(
"name" => __( 'Excursions', '' ),
"singular_name" => __( 'Excursion', '' ),
"menu_name" => __( 'Excursions', '' ),
"all_items" => __( 'All Excursions', '' ),
"add_new" => __( 'Add Excursion', '' ),
"add_new_item" => __( 'Add New Excursion', '' ),
"edit" => __( 'Edit', '' ),
"edit_item" => __( 'Edit Excursion', '' ),
"new_item" => __( 'New Excursion', '' ),
"view" => __( 'View', '' ),
"view_item" => __( 'View Excursion', '' ),
"search_items" => __( 'Search Excursions', '' ),
);
$args = array(
"label" => __( 'Excursions', '' ),
"labels" => $labels,
"description" => "Hotels also reference \'Hotels\'",
"public" => true,
"show_ui" => true,
"show_in_rest" => false,
"rest_base" => "",
"has_archive" => false,
"show_in_menu" => true,
"exclude_from_search" => false,
"capability_type" => "post",
"map_meta_cap" => true,
"hierarchical" => false,
"rewrite" => array( "slug" => "excursion", "with_front" => true ),
"query_var" => true,
"menu_position" => 20,"menu_icon" => "dashicons-tickets-alt",
"supports" => array( "title", "comments", "revisions", "thumbnail", "page-attributes" ),
"taxonomies" => array( "holiday-type" ),
);
register_post_type( "excursion", $args );
$labels = array(
"name" => __( 'Hotels', '' ),
"singular_name" => __( 'Hotel', '' ),
"menu_name" => __( 'Hotels', '' ),
"all_items" => __( 'All Hotels', '' ),
"add_new" => __( 'Add Hotel', '' ),
"add_new_item" => __( 'Add New Hotel', '' ),
"edit" => __( 'Edit', '' ),
"edit_item" => __( 'Edit Hotel', '' ),
"new_item" => __( 'New Hotel', '' ),
"view" => __( 'View', '' ),
"view_item" => __( 'View Hotel', '' ),
"search_items" => __( 'Search Hotels', '' ),
);
$args = array(
"label" => __( 'Hotels', '' ),
"labels" => $labels,
"description" => "Hotels also reference \'Excursions\'.",
"public" => true,
"show_ui" => true,
"show_in_rest" => false,
"rest_base" => "",
"has_archive" => false,
"show_in_menu" => true,
"exclude_from_search" => false,
"capability_type" => "post",
"map_meta_cap" => true,
"hierarchical" => false,
"rewrite" => array( "slug" => "hotel", "with_front" => true ),
"query_var" => true,
"menu_position" => 21,"menu_icon" => "dashicons-store",
"supports" => array( "title", "comments", "revisions", "thumbnail", "page-attributes" ),
"taxonomies" => array( "holiday-type" ),
);
register_post_type( "hotel", $args );
$labels = array(
"name" => __( 'Offers', '' ),
"singular_name" => __( 'Offer', '' ),
"menu_name" => __( 'Offers', '' ),
"all_items" => __( 'All Offers', '' ),
"add_new" => __( 'Add Offer', '' ),
"add_new_item" => __( 'Add New Offer', '' ),
"edit" => __( 'Edit', '' ),
"edit_item" => __( 'Edit Offer', '' ),
"new_item" => __( 'New Offer', '' ),
"view" => __( 'View', '' ),
"view_item" => __( 'View Offer', '' ),
"search_items" => __( 'Search Offers', '' ),
);
$args = array(
"label" => __( 'Offers', '' ),
"labels" => $labels,
"description" => "An \'Offer\' can be made up of Hotels & Excursions",
"public" => true,
"show_ui" => true,
"show_in_rest" => false,
"rest_base" => "",
"has_archive" => false,
"show_in_menu" => true,
"exclude_from_search" => false,
"capability_type" => "post",
"map_meta_cap" => true,
"hierarchical" => false,
"rewrite" => array( "slug" => "offer", "with_front" => true ),
"query_var" => true,
"menu_position" => 22,"menu_icon" => "dashicons-smiley",
"supports" => array( "title", "comments", "revisions", "thumbnail", "page-attributes" ),
"taxonomies" => array( "holiday-type" ),
);
register_post_type( "offer", $args );
$labels = array(
"name" => __( 'Banners', '' ),
"singular_name" => __( 'Banner', '' ),
"menu_name" => __( 'Banners', '' ),
"all_items" => __( 'All Banners', '' ),
"add_new" => __( 'Add Banner', '' ),
"add_new_item" => __( 'Add New Banner', '' ),
"edit" => __( 'Edit', '' ),
"edit_item" => __( 'Edit Banner', '' ),
"new_item" => __( 'New Banner', '' ),
"view" => __( 'View', '' ),
"view_item" => __( 'View Banner', '' ),
"search_items" => __( 'Search Banner', '' ),
);
$args = array(
"label" => __( 'Banners', '' ),
"labels" => $labels,
"description" => "Banners shown in various locations across the website",
"public" => true,
"show_ui" => true,
"show_in_rest" => false,
"rest_base" => "",
"has_archive" => false,
"show_in_menu" => true,
"exclude_from_search" => false,
"capability_type" => "post",
"map_meta_cap" => true,
"hierarchical" => false,
"rewrite" => array( "slug" => "banner", "with_front" => true ),
"query_var" => true,
"menu_icon" => "dashicons-welcome-widgets-menus",
"supports" => array( "title" ),
"taxonomies" => array( "banner-locations" ),
);
register_post_type( "banner", $args );
$labels = array(
"name" => __( 'Tours', '' ),
"singular_name" => __( 'Tour', '' ),
"menu_name" => __( 'Tours', '' ),
"all_items" => __( 'All Tours', '' ),
"add_new" => __( 'Add New', '' ),
"add_new_item" => __( 'Add New Tour', '' ),
"edit" => __( 'Edit', '' ),
"edit_item" => __( 'Edit Tour', '' ),
"new_item" => __( 'New Tour', '' ),
"view" => __( 'View', '' ),
"view_item" => __( 'View Tour', '' ),
"search_items" => __( 'Search Tours', '' ),
);
$args = array(
"label" => __( 'Tours', '' ),
"labels" => $labels,
"description" => "",
"public" => true,
"show_ui" => true,
"show_in_rest" => false,
"rest_base" => "",
"has_archive" => false,
"show_in_menu" => true,
"exclude_from_search" => false,
"capability_type" => "post",
"map_meta_cap" => true,
"hierarchical" => true,
"rewrite" => array( "slug" => "tours", "with_front" => true ),
"query_var" => true,
"menu_position" => 23,"menu_icon" => "dashicons-location-alt",
"supports" => array( "title", "comments", "revisions", "thumbnail" ),
"taxonomies" => array( "holiday-type" ),
);
register_post_type( "tours", $args );
// End of cptui_register_my_cpts()
}
add_action( 'init', 'cptui_register_my_taxes' );
function cptui_register_my_taxes() {
$labels = array(
"name" => __( 'Holiday Types', '' ),
"singular_name" => __( 'Holiday Type', '' ),
"menu_name" => __( 'Holiday Types', '' ),
"all_items" => __( 'All Holiday Types', '' ),
"edit_item" => __( 'Edit Holiday Type', '' ),
"view_item" => __( 'View Holiday Type', '' ),
"update_item" => __( 'Update Holiday Type', '' ),
"add_new_item" => __( 'Add New Holiday Type', '' ),
"new_item_name" => __( 'New Holiday Type', '' ),
"parent_item" => __( 'Parent Holiday Type', '' ),
"parent_item_colon" => __( 'Parent Holiday Type:', '' ),
"search_items" => __( 'Search Holiday Type', '' ),
"popular_items" => __( 'Popular Holiday Types', '' ),
"add_or_remove_items" => __( 'Add or Remove Holiday Types', '' ),
);
$args = array(
"label" => __( 'Holiday Types', '' ),
"labels" => $labels,
"public" => true,
"hierarchical" => true,
"label" => "Holiday Types",
"show_ui" => true,
"query_var" => true,
"rewrite" => array( 'slug' => 'holiday-type', 'with_front' => true 'hierarchical' => true ),
"show_admin_column" => false,
"show_in_rest" => false,
"rest_base" => "",
"show_in_quick_edit" => false,
);
register_taxonomy( "holiday-type", array( "excursion", "hotel", "offer" ), $args );
// End cptui_register_my_taxes()
}
Ash Bryant
April 8, 2016 at 9:28 am
I’ve tried adding
echo '$post_type: <pre>' . $post_type . '</pre>';
but it doesn’t show anything, but if I change your code to match Colir’s version here …
// Retrieve post type based on post name
$post_type = $wpdb->get_var(
$wpdb->prepare(
'SELECT post_type FROM ' . $wpdb->posts . ' WHERE post_name = %s LIMIT 1',
$post_name
)
);
it returns as banner, so is it the query here that is the issue?
Ryan Sechrest
April 8, 2016 at 4:18 pm
Hi Ash,
I took your code, with a minor tweak in labels:
"rewrite" => array( 'slug' => 'holiday-type', 'with_front' => true 'hierarchical' => true ),
You’re missing a comma after thetrue
before'hierarchical'
.
Then I took all of my code above, with the revised version from 12/23/14, which basically is this:
function custom_pre_get_posts($query) {
global $wpdb;
if(!$query->is_main_query()) {
return;
}
$post_name = $query->get('pagename');
$result = $wpdb->get_row(
$wpdb->prepare(
'SELECT wpp1.post_type, wpp2.post_name AS parent_post_name FROM ' . $wpdb->posts . ' as wpp1 LEFT JOIN ' . $wpdb->posts . ' as wpp2 ON wpp1.post_parent = wpp2.ID WHERE wpp1.post_name = %s LIMIT 1',
$post_name
)
);
switch($result->post_type) {
case 'tours':
if ($result->parent_post_name !== '') {
$post_name = $result->parent_post_name . '/' . $post_name;
}
$query->set('services', $post_name);
$query->set('post_type', $result->post_type);
$query->is_single = true;
$query->is_page = false;
break;
}
}
I pasted it into a functions.php file on a multisite I’m running, and added multiple tours to two different sites. Each permalink had the post type removed and I saw each tour page render (using the default template).
There must be something else you have tweaked or installed that is interfering with the code above, but based on what you posted, I can confirm that it works.
Ash Bryant
April 15, 2016 at 10:42 am
Odd. Could domain mapping be causing the issue do you think?
Ryan Sechrest
April 15, 2016 at 11:10 am
Is that a plugin or are you doing it manually? The site network I tested it on actually uses a top-level domains for each site (as apposed to subdomain or subdirectory), so that does work as well.
Ash Bryant
April 15, 2016 at 11:30 am
Bugger.
It’s a plugin, this one https://wordpress.org/plugins/wordpress-mu-domain-mapping/
If it’s not that, then the only other thing I can think of that might be causing the issue is the WPML plugin that allow the client to translate their website.
Ryan Sechrest
April 15, 2016 at 2:17 pm
I could definitely see WPML causing issues, since it manipulates database queries. I run a network of sites with WPML, but I don’t remove post type slugs on any of those sites. You might deactivate both the domain mapping and WPML plugin to see if that resolves the issue. If so, then at least you know where to look to find a workaround.
Alex
April 26, 2016 at 9:50 am
Hi, Ryan.
Thank for you post. It’s very useful.
But I was faced with another problem. I want to change woocommerce product url. Page returns a 404 error, and inWP_Query
Object object i have
[query] => Array
(
[error] => 404
)
No relevant information. What it can be? It looks like the function is executed after construction of the post.
I would be grateful for any advice
Ryan Sechrest
April 26, 2016 at 11:38 am
Hi Alex! I don’t use WooCommerce, so I don’t have any information that would be helpful here. I will say that my tutorial is for changing the URL for post types that you register. Changing the URLs in post types registered by a third-party plugin might have unintended consequences.
Ajay
January 19, 2017 at 1:47 pm
Awesome. One of the few solutions that work to get the URL’s right !!!! Thank you
Leo
February 11, 2018 at 8:54 am
Hi Ryan,
I am using WP 4.9.2 and I had to change:$post_name = $query->get(‘pagename’);
to$post_name = $query->query[‘name’];
because the first one was always empty.
Maybe it could help somebody…
Viktor Ormanow
April 3, 2020 at 1:18 am
Yeah, same issue on WP. Plug-ins do help but slow down the page speed.
Forbes Robertson
February 23, 2021 at 9:02 am
Greetings!
I hope that someone still keeps an eye on this post.
I have implemented the code above and I am able to rewrite the URL fromdomainname.com/commerce/post-name
todomainname.com/post-name
I gotta say this works excellent.
I am running into a problem. Everything works fine if I initially publish a post… the slug gets create and saved and the post is viewable.
The “Edit Permalink” button is gone.
When I save as draft, the slug does not get written and the permalink is empty.
Here is my code:
DECLARE CUSTOM POST TYPE
<?php
function custom_post_type_commerce() {
$labels = array(
'name' => _x( 'Commerce', 'post type general name' ),
'singular_name' => _x( 'Commerce', 'post type singular name' ),
'add_new' => _x( 'Add New', 'Commerce' ),
'add_new_item' => __( 'Add New Commerce' ),
'edit_item' => __( 'Edit Commerce' ),
'new_item' => __( 'New Commerce' ),
'all_items' => __( 'All Commerce' ),
'view_item' => __( 'View Commerce' ),
'search_items' => __( 'Search Commerce' ),
'not_found' => __( 'No Commerce found' ),
'not_found_in_trash' => __( 'No Commerce found in the Trash' ),
'parent_item_colon' => '',
'menu_name' => 'Commerce'
);
$supports = array(
'author',
'comments',
'custom-fields',
'editor',
'excerpt',
'page-attributes',
'revisions',
'thumbnail',
'title'
);
$args = array(
'capability_type' => 'page',
'has_archive' => true,
'hierarchical' => true,
'labels' => $labels,
'public' => true,
'rewrite' => false,
'show_ui' => true,
'exclude_from_search' => true,
'taxonomies' => array('category', 'post_tag'),
'supports' => $supports,
);
register_post_type( 'commerce', $args );
}
add_action( 'init', 'custom_post_type_commerce' );
REWRITE CODE
add_action( 'post_type_link', 'custom_post_type_link', 10, 3 );
function custom_post_type_link($permalink, $post, $leavename) {
if ( !gettype( $post ) == 'post' ) {
return $permalink;
}
switch ( $post->post_type ) {
case 'commerce':
$permalink = get_home_url() . '/' . $post->post_name . '/';
break;
}
return $permalink;
}
add_action( 'pre_get_posts', 'custom_pre_get_posts' );
function custom_pre_get_posts($query) {
global $wpdb;
if( !$query->is_main_query() ) {
return;
}
$post_name = $query->get( 'pagename' );
$result = $wpdb->get_row(
$wpdb->prepare(
'SELECT wpp1.post_type, wpp2.post_name AS parent_post_name FROM ' . $wpdb->posts . ' as wpp1 LEFT JOIN ' . $wpdb->posts . ' as wpp2 ON wpp1.post_parent = wpp2.ID WHERE wpp1.post_name = %s LIMIT 1',
$post_name
)
);
if( !empty( $result->post_type ) ) {
switch( $result->post_type ) {
case 'commerce':
if ( $result->parent_post_name !== '' ) {
$post_name = $result->parent_post_name . '/' . $post_name;
}
$query->set( 'commerce', $post_name );
$query->set( 'post_type', $result->post_type );
$query->is_single = true;
$query->is_page = false;
break;
}
}
return $query;
}
Ryan Sechrest
February 23, 2021 at 11:44 am
Hi Forbes, it looks like WordPress changed a few things since I published this. You could return the default permalink unless the post is published. So something like:
function custom_post_type_link($permalink, $post, $leavename) {
if ( !gettype( $post ) == 'post' ) {
return $permalink;
}
if ( $post->post_type !== 'commerce' ) {
return $permalink;
}
if ( $post->post_status !== 'publish') {
return $permalink;
}
return get_home_url() . '/' . $post->post_name . '/';
}
Forbes Robertson
February 23, 2021 at 2:39 pm
Thank you Ryan. I will see if I can get this to work as expected.